Understanding Garbage Collection Hooks
What is a Garbage Collection Hook?
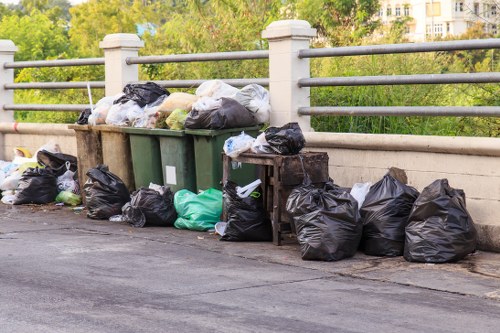
Garbage Collection Hooks are essential tools in programming, especially in languages that manage memory automatically. They allow developers to execute custom code when the garbage collector runs, providing better control over memory management.
In many programming environments, garbage collection is an automatic process that frees up memory by removing objects that are no longer in use. However, sometimes developers need to perform specific actions during this process, and that's where hooks come into play.
By utilizing garbage collection hooks, you can optimize your application's performance, manage resources more efficiently, and prevent memory leaks. This article explores the ins and outs of garbage collection hooks, their benefits, and how to implement them effectively.
How Garbage Collection Works
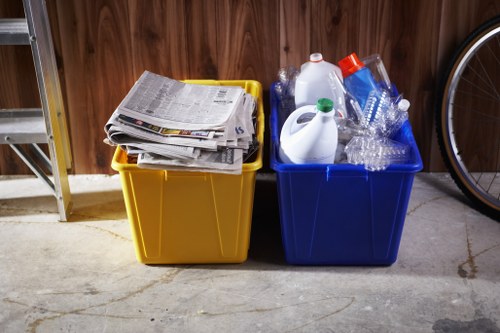
Understanding garbage collection hooks starts with knowing how garbage collection itself operates. Garbage collection is the process by which a programming language automatically reclaims memory occupied by objects that are no longer needed.
The garbage collector periodically scans memory to identify objects that are unreachable or no longer referenced by the application. Once identified, these objects are removed, freeing up memory for future use.
This automatic memory management helps prevent common issues like memory leaks, where unused memory is not released, leading to wasted resources and potential application crashes.
Why Use Garbage Collection Hooks?
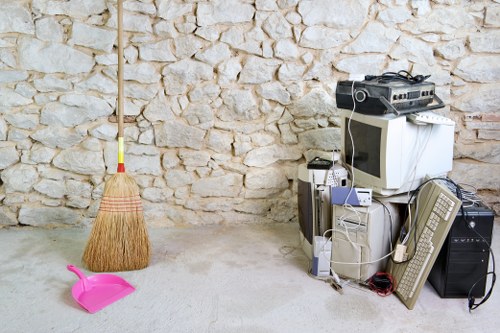
While automatic garbage collection is beneficial, there are scenarios where you might need more control. Garbage collection hooks allow you to execute custom code during the garbage collection process, offering several advantages:
- Resource Management: Release or reallocate resources when garbage collection occurs.
- Performance Optimization: Measure and analyze memory usage patterns.
- Custom Logging: Track garbage collection events for debugging and monitoring.
- Enhanced Control: Implement custom cleanup procedures tailored to your application's needs.
Implementing Garbage Collection Hooks
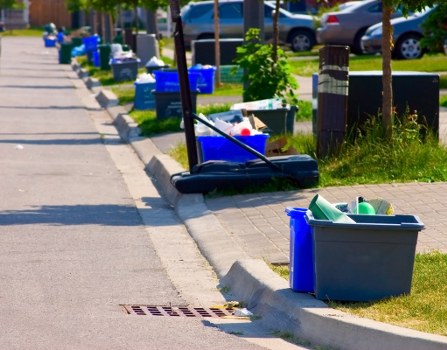
Implementing garbage collection hooks varies depending on the programming language and environment you're using. Let's look at some common approaches:
- Java: In Java, you can use the
java.lang.ref.Cleaner
class to perform actions when an object is garbage collected. - C#: The
Finalize
method can be overridden to execute code during garbage collection. - Python: The
__del__
method allows you to define cleanup behavior when an object is about to be destroyed.
Best Practices for Using Hooks
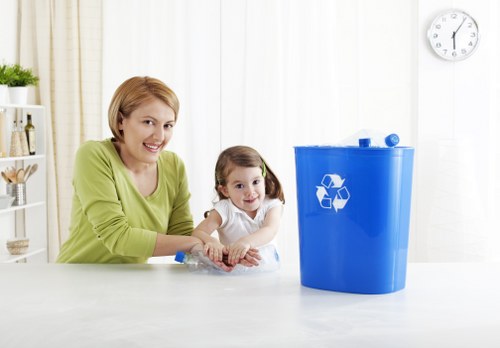
To effectively use garbage collection hooks, consider the following best practices:
- Avoid Heavy Operations: Keep the code in hooks lightweight to prevent slowing down the garbage collector.
- Handle Exceptions: Ensure that your hook code handles exceptions gracefully to avoid disrupting the garbage collection process.
- Minimize Dependencies: Reduce dependencies within your hook code to maintain performance and reliability.
- Test Thoroughly: Rigorously test your hooks to ensure they behave as expected during garbage collection events.
Local Relevance: Garbage Collection in Hook Areas
Understanding how garbage collection hooks function can be particularly beneficial in the nearby areas of Hook. Let's explore some of these areas:
- Brooklands: Known for its tech startups, Brooklands often leverage advanced garbage collection techniques to optimize performance.
- Fordwick: Home to several software development firms, Fordwick emphasizes efficient memory management in their applications.
- Holtville: With a focus on sustainability, Holtville integrates garbage collection hooks to manage resources responsibly.
- Lyndon: Lyndon's growing tech community benefits from the enhanced control provided by garbage collection hooks.
- Marston: Developers in Marston use garbage collection hooks to improve application stability and performance.
- Newton: Newton's software engineers rely on hooks to maintain optimal memory usage in their projects.
- Oakridge: Oakridge integrates garbage collection hooks into their systems to prevent memory leaks and ensure efficiency.
- Peaksville: Peaksville developers implement hooks to monitor and manage memory effectively.
- Quinton: Quinton's tech enterprises use garbage collection hooks to enhance their application's robustness.
- Riverside: Riverside's programming community values the control and optimization provided by garbage collection hooks.
Challenges and Considerations
While garbage collection hooks offer numerous benefits, there are also challenges to consider:
- Complexity: Implementing hooks adds complexity to your codebase, which can increase maintenance efforts.
- Performance Overhead: Improperly implemented hooks can introduce performance overhead, counteracting the benefits of garbage collection.
- Language Limitations: Not all programming languages support garbage collection hooks, limiting their applicability.
- Debugging Difficulty: Issues within hooks can be harder to trace, complicating the debugging process.
Future of Garbage Collection Hooks
The future of garbage collection hooks looks promising as programming languages continue to evolve. Advances in memory management techniques will likely lead to more sophisticated and efficient hooks, providing developers with even greater control and flexibility.
Integration with modern development tools and frameworks will also enhance the usability and functionality of garbage collection hooks, making them more accessible to a broader range of developers.
As applications become more complex and resource-intensive, the importance of effective memory management and garbage collection hooks will only grow, driving innovation in this area.
Conclusion
Garbage Collection Hooks are powerful tools that give developers enhanced control over memory management in their applications. By understanding how garbage collection works and how to implement hooks effectively, you can optimize performance, manage resources efficiently, and prevent memory-related issues.
While there are challenges to using hooks, the benefits they offer make them a valuable addition to any developer's toolkit. As technology advances, the role of garbage collection hooks will continue to expand, providing even more opportunities for optimization and control.
Frequently Asked Questions
1. What is the main purpose of a garbage collection hook?
The main purpose of a garbage collection hook is to allow developers to execute custom code during the garbage collection process, enabling better control over memory management and resource handling.
2. Can garbage collection hooks improve application performance?
Yes, when used correctly, garbage collection hooks can improve application performance by optimizing memory usage and preventing memory leaks.
3. Are garbage collection hooks supported in all programming languages?
No, garbage collection hooks are not supported in all programming languages. Their availability and implementation methods vary depending on the language and its runtime environment.
4. What are some best practices for implementing garbage collection hooks?
Best practices include keeping hook code lightweight, handling exceptions gracefully, minimizing dependencies, and thoroughly testing the hooks to ensure they perform as expected.
5. What challenges might I face when using garbage collection hooks?
Challenges include increased code complexity, potential performance overhead, language limitations, and difficulties in debugging issues within hooks.